3. Three leds¶
Mount the following electrical schematic on a breadboard.
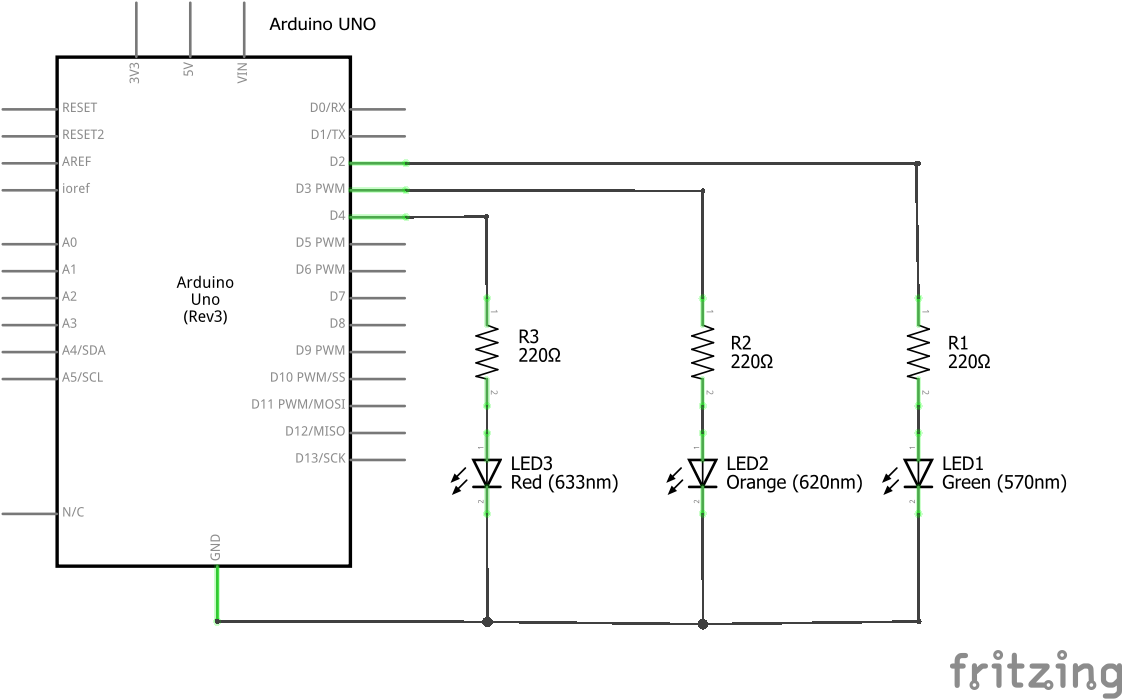
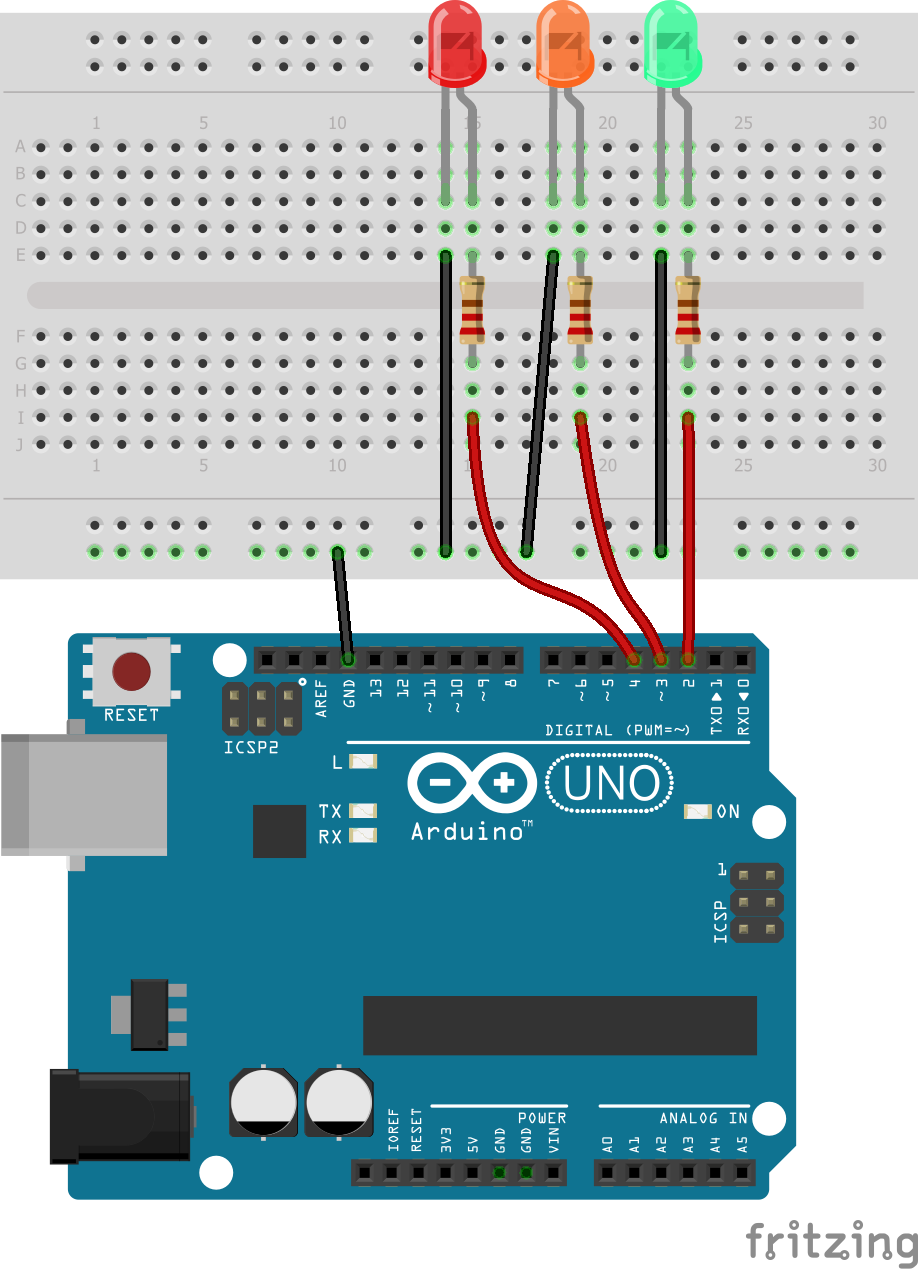
Electrical circuit in Fritzing format
Exercises¶
Upload the following program to the Arduino UNO board. All three LEDs should light up at the same time.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
// Define el pin de cada led int LED_VERDE = 2; int LED_AMBAR = 3; int LED_ROJO = 4; // Ejecuta una sola vez las siguientes instrucciones void setup() { // Conecta los tres ledes a salidas pinMode(LED_VERDE, OUTPUT); pinMode(LED_AMBAR, OUTPUT); pinMode(LED_ROJO, OUTPUT); } // Repite para siempre las siguientes instrucciones void loop() { // Enciende los tres ledes digitalWrite(LED_VERDE, HIGH); digitalWrite(LED_AMBAR, HIGH); digitalWrite(LED_ROJO, HIGH); }
Modify the previous program so that the green led lights up first, a second later the amber led and a second later the red led.
The instruction to use to wait for a second is:
1
delay(1000);
Modify the previous program so that after all the LEDs are on, they go off one by one, starting by turning off the red led and ending by turning off the green led. The time between shutdowns will be one second.
The instruction to be used to turn off an LED is:
1
digitalWrite(LED_VERDE, LOW);
Modify the program so that it works like a semaphore.
First the green led will light up for 3 seconds.
Then the green led will turn off and the amber led will light up for 1 second.
Then the amber led will turn off and the red led will light up for 3 seconds.
The sequence will repeat continuously.
Modify the previous program so that the amber led blinks three times. The on and off time will be half a second.
Perform a program with a different sequence than the previous exercises.