4. DHT11 temperature and humidity sensor¶
The DHT11 sensor is a digital sensor for temperature and relative humidity of the air. It uses digital communication with Arduino, so it is not necessary to connect to an analog pin to take the readings.
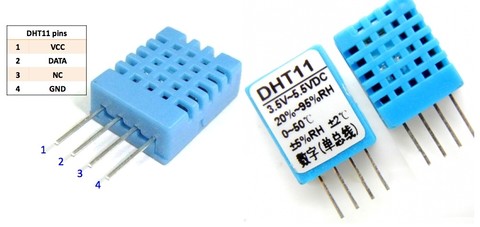
Technical specifications¶
- Supply voltage from 3 to 5 volts
- Maximum supply current 2.5 mA
- Relative humidity range 20% to 80% with 5% accuracy
- Temperature range from 0 to 50ºC with +-2ºC accuracy
- Speed of 1 measurement per second
- Size 15.5mm x 12mm x 5.5mm
- 4 pin connection
Arduino Library¶
Connection scheme¶
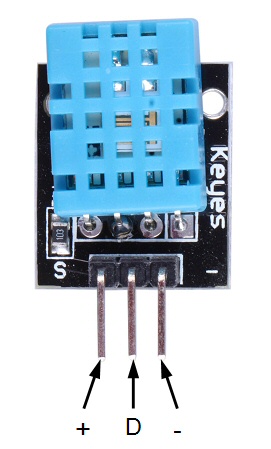
Exercises¶
The following program sends through the serial port the relative humidity and the temperature measured by the DHT11 sensor.
Upload the program to the Arduino and display the values on the serial monitor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
// // Test de temperatura y humedad // #include <dht11.h> dht11 DHT11; #define DHT11PIN 4 void setup() { Serial.begin(57600); Serial.println("DHT11 TEST PROGRAM "); int chk = DHT11.read(DHT11PIN); pinMode(2, OUTPUT); digitalWrite(2, HIGH); } void loop() { delay(1000); // Lee sensor Serial.println("\n"); Serial.print("Leyendo sensor... "); int chk = DHT11.read(DHT11PIN); switch (chk) { case DHTLIB_OK: Serial.println("Correcto"); break; case DHTLIB_ERROR_CHECKSUM: Serial.println("Error de datos"); break; case DHTLIB_ERROR_TIMEOUT: Serial.println("Error de tiempo de espera"); break; default: Serial.println("Error desconocido"); break; } // Imprimir temperatura y humedad if (chk == DHTLIB_OK) { Serial.print("Humedad (%): "); Serial.println((float)DHT11.humidity, 1); Serial.print("Temperatura (C): "); Serial.println((float)DHT11.temperature, 1); } }
Modify the previous program so that it shows the temperature measurement on the display.
Modify the previous program so that a red LED lights up if the ambient temperature exceeds 2 degrees Celsius.
Check correct operation by heating the sensor. The red led should stay on even if the temperature drops again.
Modify the above program to make a buzzer sound when the temperature is high. The buzzer will sound for a few tenths of a second every second. The instructions to use are as follows:
1 2 3
pc.buzzTone(1000); delay(20); pc.buzzTone(0);
The buzzer will stop sounding when the temperature drops again. Check correct operation by heating the sensor.
Modify the previous program so that a blue led lights up while the temperature measurement remains low.
The blue led will turn off if the measured temperature exceeds the current ambient temperature plus one degree.