9. Buzzer¶
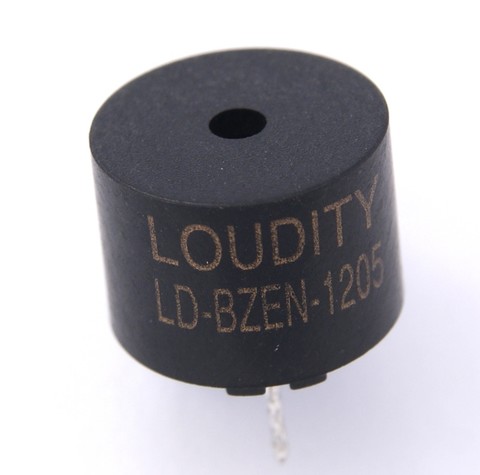
Goals¶
- Emit a tone of a certain frequency with the buzzer
- Control the sound emission time
- Emit musical notes with the buzzer
Buzzer or Buzzer emitting sounds¶
The buzzer is a small black cylindrical speaker located between the keyboard and the display. Its function is to make acoustic signals to attract attention, for this reason it has a particularly high-pitched and penetrating sound. The quality of the emitted sound is poor. The buzzer has no control over the intensity of the sound or its timbre (loudness). On the other hand, the buzzer does have the ability to reproduce different musical notes by controlling the frequency (tone) and duration (figure) of the emitted sound. This allows you to play sheet music in a simple way.
The units used to measure frequency will be hertz, or its symbol Hz.
One hertz equals one oscillation per second. Another common unit is the kilohertz or kHz which is equivalent to 1000 Hz or one thousand oscillations per second.
The units used to measure time will be milliseconds or its symbol ms
One thousand milliseconds equals to one second.
The human ear is most sensitive to the frequency range from 500 Hz (bass) to 2000 Hz (treble). This range is covered by approximately the 5th and 6th octaves (musical second and third octaves).
The buzzFreq()
function¶
-
buzzFreq
(int Frequency)¶ This function emits a sound through the buzzer with a certain frequency.
Frequency
: This parameter sets the frequency of the sound to be emitted by the buzzer. A zero frequency turns off the internal oscillator and keeps the buzzer silent. The valid frequency range is from 15 hertz to 32767 hertz.
The human ear can perceive sounds of a frequency up to 20,000 hertz in the best of cases. Above 20,000 Hz, ultrasound begins, which humans cannot perceive. As a person ages, their sensitivity to high frequencies decreases, so that, in practice, most people are unable to distinguish sounds with frequencies above 16,000 Hz.
In the example below, pressing button 1 will cause the buzzer to sound at 2000 Hz for 62 milliseconds and remain off for 62 milliseconds. The sound will repeat as long as pad 1 is held down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | // Emite un tono de 2000 hercios al presionar el pulsador 1
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Si se presiona el pulsador 1
if (pc.keyPressed(1)) {
pc.buzzFreq(2000); // Emite un sonido de 2000 Hz
delay(62); // durante 62 milisegundos
pc.buzzFreq(0); // Apaga el generador de sonido
delay(62); // durante 62 milisegundos
}
}
|
The buzzTone()
function¶
-
buzzTone
(int Tone)¶ This function is similar to the
buzzFreq()
function, it produces a sound of a frequency determined by theTone
parameter.Tone
: Musical note that will sound on the buzzer. The note can be expressed with a number from 0 to 127 or with a constant. Note 0 (Silence
) is special and serves to silence the sound generator.The following table represents the constants, the equivalent value and the notes they represent for the first musical octave (fourth octave in scientific notation).
Constant Worth Frequency Scientific note Note C4 49 261hz C4 Do C_4 fifty 277hz C#4 C sharp (C#) Re4 51 294hz D4 Re Re_4 52 311hz D#4 D sharp (D#) Mi4 53 330hz E4 My Fa4 54 349hz F4 Fa Fa_4 55 370hz F#4 F sharp (F#) Sun4 56 392hz G4 Sun Sun_4 57 415hz G#4 G sharp (G#) The4 58 440hz A4 The The_4 59 466hz A#4 A sharp (A#) Yes4 60 494hz B4 Yeah The rest of the octaves have the same denomination for the notes, changing only the final number to designate the octave. To change an octave, you can add or subtract the number 12:
C4
+ 12 =C5
C4
- 12 =C3
The following table shows the value and frequency of the Do note of each of the octaves:
Constant Worth Frequency Scientific note Note Silence 0 0hz No sound (silence) Do0 1 16.35hz C0 C sub-octave C1 13 32.70hz C1 C contra-octave C2 25 65.41hz C2 C great eighth C3 37 130.8hz C3 C Little octave C4 49 261.6hz C4 C Eighth Prime C5 61 523.2hz C5 C eighth second C6 73 1046hz C6 C Octave third C7 85 2093hz C7 C Eighth Fourth C8 97 4186hz C8 C Eighth Fifth C9 109 8372hz C9 C eighth sixth C10 121 16744hz C10 C eighth seventh Fa10 127 23679hz F10 F Eighth seventh
In the following example, pressing pad 1 will sound a lower note, and pressing pad 2 will sound a higher note. The initial note will be the A
of the first octave (A4
), which is the tone that is usually used to tune instruments.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | // Emite una nota más grave al presionar el pulsador 1
// Emite una nota más aguda al presionar el pulsador 2
#include <Wire.h>
#include <PC42.h>
int nota;
void setup() {
pc.begin(); // Inicializar el módulo PC42
nota = La4;
}
void loop() {
// Muestra la nota actual en el display
pc.dispNum(nota);
// Si se presiona el pulsador 1
if (pc.keyPressed(1)) {
// Calcular una nota más grave
nota = nota - 1;
if (nota < 1)
nota = 1;
pc.buzzTone(nota); // Emite una nota musical
delay(100); // durante 0.1 segundos
pc.buzzTone(0); // Apaga el generador de sonido
delay(500); // durante 0.5 segundos
}
// Si se presiona el pulsador 2
if (pc.keyPressed(2)) {
nota = nota + 1; // Calcular una nota más aguda
if (nota > 127)
nota = 127;
pc.buzzTone(nota); // Emite una nota musical
delay(100); // durante 0.1 segundos
pc.buzzTone(0); // Apaga el generador de sonido
delay(500); // durante 0.5 segundos
}
}
|
The following example program plays the happy birthday song each time pad 1 is pressed. The program is flexible and allows you to change the tempo faster or slower by changing the duration of the shortest note in the 'tempo' variable. ' on line 15). You can also change the octave of the song up or down by changing the value of the 'octave' variable on line 18.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | // Al presionar el pulsador 1, suena la canción de 'cumpleaños feliz'
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
int tempo;
int octave;
// Establece el tempo, en milisegundos, para la nota más corta
tempo = 120;
// Establece la octava. Hay 12 tonos y semitonos por cada octava
octave = 4 * 12;
// Apagar los sonidos
pc.buzzTone(0);
// Esperar mientras no se presione el pulsador 1
while(pc.keyPressed(1) == 0);
// Canción de cumpleaños feliz
// Primera columna = notas musicales (tono)
// Segunda columna = duración de cada nota (figura musical)
pc.buzzTone(octave + Do0); delay(3 * tempo);
pc.buzzTone(0); delay(10);
pc.buzzTone(octave + Do0); delay(1 * tempo);
pc.buzzTone(octave + Re0); delay(4 * tempo);
pc.buzzTone(octave + Do0); delay(4 * tempo);
pc.buzzTone(octave + Fa0); delay(4 * tempo);
pc.buzzTone(octave + Mi0); delay(8 * tempo);
pc.buzzTone(octave + Do0); delay(3 * tempo);
pc.buzzTone(0); delay(10);
pc.buzzTone(octave + Do0); delay(1 * tempo);
pc.buzzTone(octave + Re0); delay(4 * tempo);
pc.buzzTone(octave + Do0); delay(4 * tempo);
pc.buzzTone(octave + Sol0); delay(4 * tempo);
pc.buzzTone(octave + Fa0); delay(8 * tempo);
pc.buzzTone(octave + Do0); delay(3 * tempo);
pc.buzzTone(0); delay(10);
pc.buzzTone(octave + Do0); delay(1 * tempo);
pc.buzzTone(octave + Do1); delay(4 * tempo);
pc.buzzTone(octave + La0); delay(4 * tempo);
pc.buzzTone(octave + Fa0); delay(4 * tempo);
pc.buzzTone(octave + Mi0); delay(4 * tempo);
pc.buzzTone(octave + Re0); delay(8 * tempo);
pc.buzzTone(octave + La_0); delay(3 * tempo);
pc.buzzTone(0); delay(10);
pc.buzzTone(octave + La_0); delay(1 * tempo);
pc.buzzTone(octave + La0); delay(4 * tempo);
pc.buzzTone(octave + Fa0); delay(4 * tempo);
pc.buzzTone(octave + Sol0); delay(4 * tempo);
pc.buzzTone(octave + Fa0); delay(8 * tempo);
}
|
The buzzPlay()
function¶
-
buzzPlay
(int Tone, int milliseconds)¶ This function allows the buzzer to sound one or more musical notes for a certain time. The function parameters are as follows:
Tone
: Musical note that will sound on the buzzer. For more details, see :ref:buzzTonemilliseconds
: Time in milliseconds that the musical note will sound. It must be in a range from 1 to 2000.The function is executed immediately and the Arduino program continues with the next instruction while the buzzer sounds. This allows you to instantly send up to 16 notes, which are memorized and sounded one by one. This function allows a sheet music to play while the Arduino program continues to run.
-
int
buzzPlay
()¶ The
buzzPlay()
function with no arguments returns the number of notes that have not yet finished sounding in the score sent to the buzzer. Score memory can store up to 16 grades. If more notes are sent, the last ones sent will not be stored. If it is necessary to send more than 16 notes, you must first wait until there is free space in the score memory.This function returns zero if all notes have finished playing.
Example program that sounds a wake-up alarm using the buzzPlay()
function to send the notes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | // Al presionar el pulsador 1, suena una alarma de despertador
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Esperar mientras no se presione el pulsador 1
while(pc.keyPressed(1) == 0);
// Enviar las notas de una alarma de despertador
pc.buzzPlay(Do7, 62);
pc.buzzPlay(Silence, 62);
pc.buzzPlay(Do7, 62);
pc.buzzPlay(Silence, 62);
pc.buzzPlay(Do7, 62);
pc.buzzPlay(Silence, 62);
pc.buzzPlay(Do7, 62);
pc.buzzPlay(Silence, 566);
// Esperar a que suenen todas las notas
while (pc.buzzPlay());
}
|
Example program playing clock music using the buzzPlay()
function. In this example, the display shows the number of notes that have not yet finished playing.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | // Al presionar el pulsador 1, suena un carrillón y el
// display muestra el número de notas que aún no han
// terminado de sonar.
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Esperar mientras no se presione el pulsador 1
while(pc.keyPressed(1) == 0);
// Enviar la partitura de carrillón
pc.buzzPlay(La4, 500);
pc.buzzPlay(Fa4, 500);
pc.buzzPlay(Sol4, 500);
pc.buzzPlay(Do4, 1000);
pc.buzzPlay(Silence, 10);
pc.buzzPlay(Do4, 500);
pc.buzzPlay(Sol4, 500);
pc.buzzPlay(La4, 500);
pc.buzzPlay(Fa4, 1000);
// Esperar hasta que suene toda la partitura
// Mostrar el número de notas restantes
int notas;
do {
notas = pc.buzzPlay();
pc.dispNum(notas);
} while (notas > 0);
}
|
The functions buzzOff()
and buzzOn()
¶
-
buzzOff
()¶ Disconnect the sound generator from the buzzer. As a result, the buzzer stops making sound. If the buzzer is currently running, it will continue to run, so if the buzzer is turned on again with the
buzzOn()
function, the buzzer will sound again.
-
buzzOn
()¶ Connect the sound generator with the buzzer. If at that time the sound generator is generating a tone, the buzzer will start to sound.
Example of BuzzOn and BuzzOff operation. The following program generates a melody that plays continuously. By pressing button 1, the sound is switched off. By pressing button 2, the sound will reconnect.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | // Genera una melodía que suena de forma contínua
// Presionando el pulsador 1, el sonido se desconecta.
// Presionando el pulsador 2, el sonido volverá a conectarse.
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Tocar una melodía
pc.buzzPlay(Sol5, 500);
pc.buzzPlay(La5, 500);
pc.buzzPlay(Fa5, 500);
pc.buzzPlay(Fa4, 500);
pc.buzzPlay(Do5, 1000);
pc.buzzPlay(0, 500);
delay(500);
// Espera hasta que termine de sonar la melodía.
// Durante ese tiempo desconecta o conecta el sonido
// si se presionan los pulsadores 1 y 2
while(1) {
if (pc.keyPressed(1)) // Si se presiona el pulsador 1
pc.buzzOff(); // desconecta el sonido.
if (pc.keyPressed(2)) // Si se presiona el pulsador 2
pc.buzzOn(); // conecta el sonido.
if (pc.buzzPlay() == 0) // Si la nota ha terminado
return; // termina la espera.
}
}
|
The buzzBegin()
function¶
-
buzzBegin
()¶ This function initializes the sound generating system. Connects the sound generator to the buzzer and clears the memory of musical notes sent by the
buzzPlay()
function. When executing this function, the buzzer stops emitting sound and is ready to emit a new tone when it receives the order.It is not necessary to call this function at the beginning of every program because the more general
begin
function already initializes all systems, including the buzzer.
Sound intensity¶
The intensity of the sound emitted by the buzzer is not the same for all frequencies. At low frequencies, the intensity of the emitted sound is lower. As the frequency is increased, the intensity of the sound increases.
At around 2000 Hz the buzzer resonates and produces a much higher intensity sound than at other frequencies. The typical sound of alarm clocks has this tone. The frequency of 2000 Hz is between a Bi
note of the 3rd octave (Bi6
= 1975.53 Hz) and a Do
note of the 4th octave (Do7
= 2093.00Hz). This is a fairly high tone that the human ear perceives very well because it is in the zone of greatest hearing sensitivity, from 500 Hz to 2000 Hz.
Around 4000 Hz the buzzer also resonates producing a louder sound, but at this frequency the human ear is less sensitive than at the 2000 Hz frequency and the sound is not perceived as loud.
Frequency precision and accuracy¶
The precision of the frequency has an error of +-1%. This means that the frequency actually obtained always deviates towards the bass or towards the treble by some amount. Most of the frequencies have a deviation of less than 0.4%, while only some specific frequencies have a deviation of up to 1%. The frequency deviation is always the same for the same tone. This error can be perceived as a small detuning in the frequency. A well-trained ear perceives frequency differences of up to 0.2%.
The accuracy error of the frequency depends on the calibration that has been performed. This error produces an equal frequency variation at all emitted frequencies. The accuracy error depends on the temperature and the operating time of the panel and is around +-2%. This error will not be noticeable when making several sounds, because it affects all frequencies equally and the ear only detects notes that are out of tune if one frequency deviates from another. However, if two panels try to output a sound of the same frequency, a difference in frequency can be distinguished between them due to accuracy error.
Exercises¶
Change the following program to sound the beep of an alarm clock. The beep sequence shall be four 2000 Hertz beeps each lasting 64 milliseconds with a 64 millisecond blank space after each beep. At the end of the sequence, a time of 500 milliseconds must be waited without sound.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
// Al presionar el pulsador 1, suena una canción. // Versión con tabla de datos (array) #include <Wire.h> #include <PC42.h> // Canción // Primera columna: nota musical, silencio (0) o // pausa entre notas iguales (-1) // Segunda columna: Duración de la nota (en tempos) char song[] = { La4, 4, Fa4, 4, Sol4, 4, Do4, 8, -1, Do4, 4, Sol4, 4, La4, 4, Fa4, 8, }; void setup() { pc.begin(); // Inicializar el módulo PC42 } void loop() { int tempo, octave; char i, note, figure; // Establece el tempo, en milisegundos, para la nota más corta tempo = 125; // Establece el desplazamiento de octava. // +12 = aumenta una octava. -12 = disminuye una octava. octave = 0; // Apagar los sonidos pc.buzzTone(0); // Esperar mientras no se presione el pulsador 1 while(pc.keyPressed(1) == 0); // Tocar la canción almacenada i = 0; while(i < sizeof(song)) { note = song[i]; i = i + 1; if (note == -1) { // suena una pausa entre notas iguales pc.buzzTone(0); delay(10); } else { // suena una nota musical figure = song[i]; i = i + 1; pc.buzzTone(note + octave); delay(figure * tempo); } } }
The following program plays the score stored in the
song
array, the music of a chime. Change the sheet music for another song.1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
// Al presionar el pulsador 1, suena una canción. // Versión con tabla de datos (array) #include <Wire.h> #include <PC42.h> // Canción // Primera columna: nota musical, silencio (0) o // pausa entre notas iguales (-1) // Segunda columna: Duración de la nota (en tempos) char song[] = { La4, 4, Fa4, 4, Sol4, 4, Do4, 8, -1, Do4, 4, Sol4, 4, La4, 4, Fa4, 8, }; void setup() { pc.begin(); // Inicializar el módulo PC42 } void loop() { int tempo, octave; char i, note, figure; // Establece el tempo, en milisegundos, para la nota más corta tempo = 125; // Establece el desplazamiento de octava. // +12 = aumenta una octava. -12 = disminuye una octava. octave = 0; // Apagar los sonidos pc.buzzTone(0); // Esperar mientras no se presione el pulsador 1 while(pc.keyPressed(1) == 0); // Tocar la canción almacenada i = 0; while(i < sizeof(song)) { note = song[i]; i = i + 1; if (note == -1) { // suena una pausa entre notas iguales pc.buzzTone(0); delay(10); } else { // suena una nota musical figure = song[i]; i = i + 1; pc.buzzTone(note + octave); delay(figure * tempo); } } }
'The Cockroach' Notes:
Ce4 Do4 Do4 F4 A4
Ce4 Do4 Do4 F4 A4
F4 F4 Mi4 Mi4 Ke4 Ke4 Do4
C4 C4 C4 E4 G4
C4 C4 C4 E4 G4
C5 Re5 C5 B4 A4 G4 F4
'Happy Birthday' Notes:
C4 C4 K4 C4 F4 E4
C4 C4 Ke4 C4 G4 F4
C4 C4 C5 A4 F4 Mi4 Re4
C5 C5 A4 F4 G4 F4
To each of the previous notes, the time of each note (figure) must be added to complete the score. This time will be 200, 400 or 800 milliseconds.