7. Buttons and Counters¶
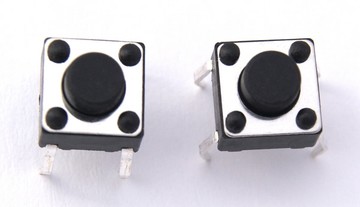
Goals¶
- Read the time and pulsation counters associated with a button.
- Perform actions associated with time counters.
The keyCount()
function¶
-
int
keyCount
(int keyNum)¶ This function returns the number of times a button has been pressed. The value is incremented by one immediately after the push button is pressed. If the button is held down for more than half a second, the pulse counter is increased by 10 pulses per second. If the button is held down for more than two seconds, the pulse counter is incremented by 100 pulses per second. The counter increment times and frequencies can be changed in the keyboard settings.
keyNum
: número del 1 al 6 que representa al pulsador del que se solicita su valor. El valor 0 representa a todos los pulsadores juntos.
The keyTimeOn()
function¶
-
int
keyTimeOn
(int keyNum)¶ This function returns the time in milliseconds that the button has been pressed. The maximum time you can count is 65 seconds. From that moment no more time is counted and the returned result is always the same.
keyNum
: número del 1 al 6 que representa al pulsador del que se solicita su valor. El valor 0 representa a todos los pulsadores juntos.
The keyTimeOff()
function¶
-
int
keyTimeOff
(int keyNum)¶ This function returns the time in milliseconds that the button has not been pressed. The maximum time you can count is 65 seconds. From that moment no more time is counted and the returned result is always the same.
keyNum
: número del 1 al 6 que representa al pulsador del que se solicita su valor. El valor 0 representa a todos los pulsadores juntos.
Exercises¶
Program the code needed to solve the following problems.
The following program moves a led to the right when button 2 is pressed. Modify the program so that the led also moves to the left when button 1 is pressed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
// Mueve una luz a la derecha con el pulsador 2 (Right) // El programa utiliza la función keyCount() #include <Wire.h> #include <PC42.h> int led, count; void setup() { pc.begin(); // Inicializar el módulo PC42 led = 1; // Enciende primero el led D1 pc.ledWrite(led, LED_ON); } void loop() { // Mueve a la derecha con la tecla derecha // Lee el número de pulsaciones del pulsador 'derecha' count = pc.keyCount(KEY_RIGHT); // Si el número de pulsaciones es mayor que cero if (count > 0) { // Apaga el led actual pc.ledWrite(led, LED_OFF); // Incrementa la posición del led led = led + count; // Si la posición del led es mayor que 6 // vuelve a la posición 1 if (led > 6) led = 1; // Enciende el led en la nueva posición pc.ledWrite(led, LED_ON); } }
The following program is a game to check the ability to tell time. Push button 1 must be pressed for exactly three seconds. The display shows the time error in milliseconds. If less time has been pressed, the number displayed will be negative. In case of pressing for more than three seconds, the displayed number will be positive. The player closest to zero wins.
Modify the program so that led D1 blinks once per second before starting the game. Once button 1 is pressed, LED 1 must go off so as not to give clues about the time the button must be pressed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
// Juego de medida de tiempo. // Gana el jugador que más se acerque a presionar el pulsador 1 // exactamente 3 segundos, y consiga así menor error. #include <Wire.h> #include <PC42.h> void setup() { pc.begin(); // Inicializar el módulo PC42 } void loop() { int time, points; // Espera hasta que se presione el pulsador 1 while(pc.keyPressed(1) == 0); // Espera hasta que se deje de presionar el pulsador 1 while(pc.keyPressed(1) == 1); // Lee el tiempo que ha estado presionado el pulsador 1 time = pc.keyTimeOn(1); // Calcula la puntuación = milisegundos de error points = time - 3000; if (points > 999) points = 999; if (points < -999) points = -999; // Muestra la puntuación por el display pc.dispNum(abs(points)); if (points < 0) pc.dispWrite(4, 0x40); // Signo menos delay(500); }
Modify the program that appears below, similar to the previous one. In this game the score will be higher if a button is pressed for the same time twice in a row. First the program must measure the time that button 1 has been pressed, then it must wait until it is not pressed. Finally, the second time that the button is pressed will be measured.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
// Juego de medida de tiempo. Versión de dos jugadores. // // El primer jugador presiona el pulsador 1 durante un tiempo. // En el display se muestra el tiempo presionado en milisegundos. // // El segundo jugador debe presionar el pulsador 1 exactamente el mismo tiempo. // El display muestra al final la diferencia entre los dos tiempos. // Gana el jugador que menos diferencia consiga. // // Para comenzar un nuevo intento se debe presionar el pulsador 1 // más de 2 segundos // #include <Wire.h> #include <PC42.h> int time1, time2; int points; void setup() { pc.begin(); // Inicializar el módulo PC42 } void loop() { // Comienza parpadeando el led D1 pc.ledBlink(1, 500, 500); // Espera hasta que se presione el pulsador 1 while(pc.keyPressed(1) == 0); // Cuenta el tiempo que está presionado el pulsador 1 while(pc.keyPressed(1) == 1) { time1 = pc.keyTimeOn(1); pc.dispNum(time1); } // Espera hasta que se presione el pulsador 1 while(pc.keyPressed(1) == 0); // Cuenta el tiempo que está presionado el pulsador 1 while(pc.keyPressed(1) == 1) { time2 = pc.keyTimeOn(1); pc.dispNum(time2); } // Apaga el led D1 y espera un segundo pc.ledWrite(1, LED_OFF); // Calcula la puntuación points = time1 - time2; // Muestra la puntuación por el display parpadeando while(1) { pc.dispNum(abs(points)); delay(500); pc.dispBegin(); delay(500); // Salir del bucle si se presiona el pulsador 1 if (pc.keyEvents(1, KEY_PRESSED_TIME2)) break; } // Espera hasta que no se presione el pulsador 1 while(pc.keyPressed(1) == 1); }