8. 7 segment display¶
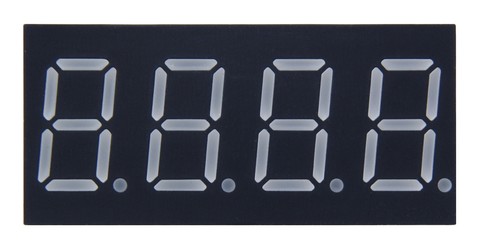
Goals¶
- Write a number on the 7-segment display.
- Write alphanumeric characters on the 7-segment display.
7 segment display¶
The display or display of seven segments is an element that allows to show numbers and also symbols and characters in a limited way. This type of display is used where good visibility is desired and has the advantage of being robust and easy to handle. 7-segment displays can be commonly found on ceramic hobs, battery chargers, audio players, microwave ovens, washing machines, watches, etc.
In this type of display, you only have to define the state of seven elements to form the desired number or letter. In other types of more complex displays, it is necessary to define the state of 35 or more points to form a number or character. The disadvantage of the 7-segment display is based on its poor ability to represent letters and symbols.
In the attached figure you can see a 7-segment display and the nomenclature of its elements.
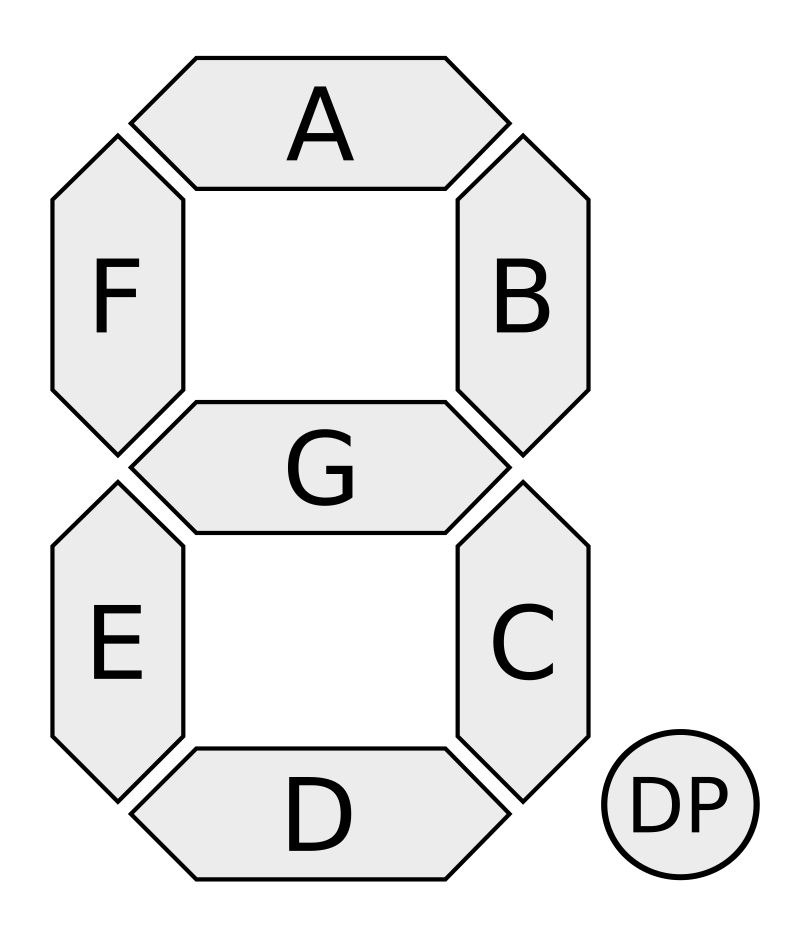
The function dispNum()
¶
-
dispNum
(int number)¶ The argument of the function
dispNum()
is a positive integer (0, 1, 2, ... 32767). This number will be displayed with four digits on the display. If the number has less than four digits, the display turns off the unused digits on the left. If the number is greater than 9999, only the right four digits are represented.Here are some display examples.
dispNum(0); -> [ 0 ] dispNum(1); -> [ 1 ] dispNum(20); -> [ 2 0 ] dispNum(124); -> [ 1 2 4 ] dispNum(2345); -> [ 2 3 4 5 ] dispNum(10321); -> [ 0 3 2 1 ]
The example below represents a number on the display that increases and decreases with buttons 3 and 4.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | // Muestra un número en el display que disminuye o aumenta
// Presionando los pulsadores 3 y 4
#include <Wire.h>
#include <PC42.h>
int num;
void setup() {
pc.begin(); // Inicializar el módulo PC42
num = 100; // Valor inicial a representar
}
void loop() {
// Actualiza el valor del número a representar
num = num + pc.keyCount(KEY_UP);
num = num - pc.keyCount(KEY_DOWN);
if (num < 0) num = num + 1000;
if (num >= 1000) num = num - 1000;
// Representa el número en el display
pc.dispNum(num);
}
|
The dispWrite()
function with two arguments¶
The dispWrite()
function allows you to write numbers and characters on the seven-segment, four-digit display. Depending on the number of arguments, the function will behave differently.
-
dispWrite
(int position, int segments)¶ When the arguments to the
dispWrite()
function are two numbers, the first represents the position of the digit to be changed and the second represents the segments to be turned on. The digit positions are, from left to right, 1 2 3 4.The one-digit segments are turned on or off with a binary number representing each one. The first (rightmost) binary digit represents segment 'a'. The second binary digit represents segment 'b' and so on up to the eighth binary digit which does not represent any segment.
For example, the binary number 0b00000001 will turn on segment 'a' and will be seen on the 7 segment display as a top bar '¯'. The binary number 0b00000110 will turn on the 'b' and 'c' segments and will be seen on the 7-segment display as the number 1. The binary number 0b01000000 will turn on the 'g' segment and will be seen on the 7-segment display as the sign less '-'.
Sometimes it will be easier to use the values already predefined in the library. Below is a list with the standard predefined values.
- Numbers: DD_0, DD_1, DD_2, DD_3, DD_4, DD_5, DD_6, DD_7, DD_8, DD_9
- Letters: DD_A, DD_b, DD_B, DD_C, DD_d, DD_E, DD_F, DD_G, DD_g, DD_H, DD_h, DD_I, DD_i, DD_J, DD_K, DD_L, DD_n, DD_ny, DD_o, DD_O, DD_P, DD_q, DD_r, DD_S, DD_t, DD_u, DD_U, DD_y, DD_Y, DD_Z
- White space: DD_SP
Custom symbols can also be created with binary numbers.
The following program rotates a bar through the top four segments of a digit.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | // Gira un segmento alrededor de los cuatro ledes superiores de un dígito
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Enciende el segmento 'a' y espera 0.1 segundos
pc.dispWrite(1, 0b00000001);
delay(100);
// Enciende el segmento 'b' y espera 0.1 segundos
pc.dispWrite(1, 0b00000010);
delay(100);
// Enciende el segmento 'g' y espera 0.1 segundos
pc.dispWrite(1, 0b01000000);
delay(100);
// Enciende el segmento 'f' y espera 0.1 segundos
pc.dispWrite(1, 0b00100000);
delay(100);
}
|
The following program rotates a bar through all the outer segments of a digit.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | // Gira un segmento alrededor de los 6 segmentos exteriores
// del primer dígito del display.
#include <Wire.h>
#include <PC42.h>
int segment;
void setup() {
pc.begin(); // Inicializar el módulo PC42
segment = 0b00000001; // El primer segmento encendido es el 'a'
}
void loop() {
// Enciende el segmento seleccionado y espera 0.100 segundos
pc.dispWrite(1, segment);
delay(100);
// Desplaza el segmento hacia uno mayor
segment = (segment << 1);
// Si se ha llegado al segmento 'g'
if (segment == 0b01000000)
// Enciende de nuevo el segmento 'a'
segment = 0b00000001;
}
|
The dispWrite()
function with four arguments¶
-
dispWrite
(int digit, int digit, int digit, int digit)¶ When the function
dispWrite()
has four arguments, each one is interpreted as the value of each digit of the seven-segment display. This is the simplest function to visualize a word on the display.
The following example program makes the word 'HELLO' appear on the display.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | // Muestra la palabra 'HOLA' en el display
#include <Wire.h>
#include <PC42.h>
void setup() {
// Inicializar el módulo PC42
pc.begin();
// Muestra la palabra 'HOLA'
pc.dispWrite(DD_H, DD_O, DD_L, DD_A);
}
void loop() {
}
|
Exercises¶
Program the code needed to solve the following problems.
Complete the following program so that it counts backwards from 10 to 0, changing its value once every second. Once the countdown is over, the red led should light up.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
// Cuenta atrás de 10 segundos #include <Wire.h> #include <PC42.h> int count; void setup() { pc.begin(); // Inicializar el módulo PC42 count = 10; while(count > 0) { // Muestra el número en el display // Espera un segundo // Reduce la variable count en una unidad } // Muestra el número en el display // Enciende el led rojo } void loop() { }
Complete the following program to make it work as an electronic dice. When pressing button 1, a number from 1 to 6 should be shown on the display.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
// Dado electrónico #include <Wire.h> #include <PC42.h> void setup() { pc.begin(); // Inicializar el módulo PC42 } void loop() { int dado; // Calcula un número aleatorio entre 1 y 6 dado = random(1, 1 + 6); // Muestra el valor por el display // Espera 50 milisegundos // Espera mientras no se presione la tecla 1 }
Show the words 'JOSE' and 'LULU' on the display, changing from one to the other every second
Show in the fourth digit an animation that consists of lighting all the segments one by one from segment 'a' to segment 'f'. When all segments are lit, they should all go out again and the sequence will start over. The waiting time between the lighting of one segment and the next will be half a second.
Design two new symbols and write a program that shows them on the display in positions 2 and 4.
Draw some weights on the display.
It shows the words 'HELLO' and a short proper name on the display. The two words must alternate every half second.
Make an original animation on the display, showing symbols or light movements.